consolePrint
This function generates a log entry in the Console log within Preview Mode.
The log entries are for debugging purposes only and do NOT appear in the output narrative.
You CANNOT see the log entries when you run the project in an external environment such as Studio Runner.
Parameters
ARGUMENT 1 (any)
A string or value to join with other arguments to produce the log entry.
ARGUMENT 2 (any)
The next string or value to join with other arguments to produce the log entry.
ARGUMENT n (any)
The next string or value to join with other arguments to produce the log entry.
Notes
Only executed calls generate a log entry.
Null values appear as "null" in the log entry.
The Console log area does not appear unless you have a call to
consolePrint
in your project. However, if none of the calls are executed — perhaps because they are in non-called scripts — the area will be empty.The Console log area can display multiple entries, but Studio truncates the content when the total length exceeds 1 million characters. A "console log truncated" message appears when this occurs.
Most log entries combine fixed text with ATL-computed values. You can combine these elements into a single argument, but the function works more efficiently when you organize them into separate arguments. Therefore:
[[consolePrint('The actual value for Sales is ', totalSales, ' and the actual value for Target is ', totalTarget)]]
is more efficient — from a project runtime perspective — than:
[[consolePrint("The actual value for Sales is [[totalSales]] and the actual value for Target is [[totalTarget]]")]]
Examples
Assume a "Describe the Table" project with this data:
ID | Month | Quarter | Year | Units Sold | Sales | |
---|---|---|---|---|---|---|
Row 1 | 1001 | Jan | Q1 | 2022 | 1564 | 25474 |
Row 2 | 1002 | Feb | Q1 | 2022 | 2577 | 48718 |
Row 3 | 1003 | Mar | Q1 | 2022 | 1814 | 32175 |
Also, assume the Main script has this content:
[[global.totalSales = totalVal(Sales); global.totalUnitsSold = totalVal(Units_Sold);'']] We sold [[precision(totalUnitsSold,-3)]] units, generating sales of [[abbreviateNumber(currencyFormat(totalSales))]]. [[consolePrint('Actual value for Units Sold = ', totalUnitsSold)]] [[consolePrint('Actual value for Total Sales = ', totalSales)]]
Clicking Preview would produce this:
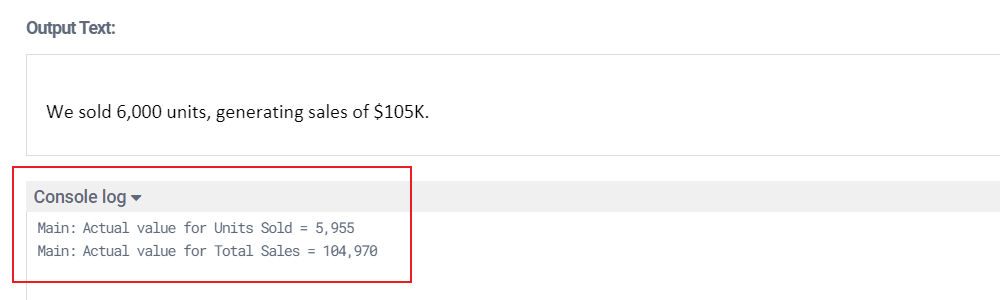
The output narrative appears in the Output Text window. The entries generated by consolePrint
appear in the Console log area directly below. Each log entry is prefixed by the relevant script name. Here, both entries are for the Main script.
In this example, each call to consolePrint
forms a standalone ATL block. You can also insert the call into an existing ATL block. This is shown in the following examples, which talk you through how to use the function in more complex projects.
Using consolePrint to retrieve values
Important
Download and open THIS EXAMPLE PROJECT to work through the example.
Open the project and preview the Main script. You should see this:
The first sentence appears mistaken: $91.5M – $84.5M = $7.0M, not $7.1M. This could be a calculation error or a round-off error — i.e. one caused by the effect of half-up rounding on large numbers. We can check quickly using
consolePrint
.Open the project's summaryAnalytics script and scroll to the end.
The script is a user-defined function that returns an ATL object. The object contains various calculated values, and these are the same values that appear (in abbreviated form) in the output narrative we looked at earlier. If we create a log entry showing the full ATL object, we can easily check the actual values against the abbreviated values in the output narrative.
Add the changes underlined in red.
Preview the Main script by clicking the Preview Main button.
You should see this:
You can now compare the abbreviated values in the narrative against the actual values calculated in the summaryAnalytics script. This shows that the discrepancy in the narrative is caused by a round-off error, not a calculation error.
Tip
You can reference individual values in an ATL object by using dot notation. For example, to get the performance value only, you might change the
consolePrint
call to:consolePrint('Actual value for performance = ', resultsObject.performance)
One last point about this example:
Here, it's necessary to make
resultsObject
the final statement because the script should return an ATL object, not a log entry.Tip
An ATL block always returns the value of its final statement. Bear this mind when adding calls to
consolePrint
.
Using consolePrint in a conditional statement
Important
Download and open THIS EXAMPLE PROJECT to work through the example.
Open the project and look at the Main script.
The first line creates a script variable (
thisYear
) that returns the current year — i.e. the year when you run the project. The second line creates a script variable (lastDataYear
) that interrogates the data's Year column and returns the most recent year with data.The next two lines comprise a simple if-else conditional. If
lastDataYear
is EQUAL TOthisYear
, Studio calls thegetSummary
function withthisYear
; else, Studio callsgetSummary
withlastDataYear
. In effect, the project generates a summary for the current or most recent year, depending on the uploaded data. You can make this clearer to other developers by usingconsolePrint
.Add the changes underlined in red.
Note that you have added the call to
consolePrint
within the ATL block that defines theelse
output. The block includes two ATL statements — the first a call toconsolePrint
, the second a call togetSummary
— separated by a semicolon.An ATL block always returns the value of its final statement; therefore, the call to
consolePrint
must precede the call togetSummary
; if they were the other way round, the conditional statement wouldn't work as intended.Still in the Main script, click Preview. You should see this:
The output text summarizes the data for 2021. The statement in the Console log area explains that there is no data for the current year (2022 at the time of writing) and confirms that 2021 is the most recent year with data.
Download THIS EXAMPLE DATASET, which includes data for 2020–2022 (inclusive).
Click Data in the left-hand menu and upload the new sample dataset.
Click Preview to run the project.
If the current year is 2022, the Console log area should be empty, as shown above.
If the current year is 2023 or beyond, a log entry will state that there is no data for the current year (e.g. 2023) and 2022 is the most recent year with data.
Note
An empty Console log area means your project includes calls to
consolePrint
but none were executed.